Mình có đoạn code này để tạo xung PWM và đang hoạt động bình thường: có xung PWM tại chân PB8 và led PD12 nháy
#include "stm32f4xx.h" // Device header void delay_ms(uint32_t u32DelayInMs); void delay_ms(uint32_t u32DelayInMs) { while (u32DelayInMs) { TIM_SetCounter(TIM6, 0); while (TIM_GetCounter(TIM6) < 1000) { } --u32DelayInMs; } } int main(void) { GPIO_InitTypeDef gpioInit; TIM_TimeBaseInitTypeDef timInit; TIM_OCInitTypeDef pwmInit; RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOD, ENABLE); gpioInit.GPIO_Mode = GPIO_Mode_OUT; gpioInit.GPIO_OType = GPIO_OType_PP; gpioInit.GPIO_Pin = GPIO_Pin_12; gpioInit.GPIO_PuPd = GPIO_PuPd_NOPULL; gpioInit.GPIO_Speed = GPIO_High_Speed; GPIO_Init(GPIOD, &gpioInit); RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM6, ENABLE); timInit.TIM_Prescaler = 84 - 1; timInit.TIM_Period = 0xFFFF; TIM_TimeBaseInit(TIM6, &timInit); TIM_Cmd(TIM6, ENABLE); /* timer 10 */ RCC_APB2PeriphClockCmd(RCC_APB2Periph_TIM10, ENABLE); timInit.TIM_Prescaler = 168 - 1; timInit.TIM_Period = 10 - 1; TIM_TimeBaseInit(TIM10, &timInit); pwmInit.TIM_OCMode = TIM_OCMode_PWM1; pwmInit.TIM_OCPolarity = TIM_OCPolarity_High; pwmInit.TIM_Pulse = 2; pwmInit.TIM_OutputState = TIM_OutputState_Enable; TIM_OC1Init(TIM10, &pwmInit); RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE); gpioInit.GPIO_Mode = GPIO_Mode_AF; gpioInit.GPIO_OType = GPIO_OType_PP; gpioInit.GPIO_Pin = GPIO_Pin_8; gpioInit.GPIO_PuPd = GPIO_PuPd_NOPULL; gpioInit.GPIO_Speed = GPIO_High_Speed; GPIO_Init(GPIOB, &gpioInit); GPIO_PinAFConfig(GPIOB, GPIO_PinSource8, GPIO_AF_TIM10); TIM_Cmd(TIM10, ENABLE); while (1) { GPIO_SetBits(GPIOD, GPIO_Pin_12); delay_ms(100); //TIM_SetCompare1(TIM10, 4); GPIO_ResetBits(GPIOD, GPIO_Pin_12); delay_ms(200); //TIM_SetCompare1(TIM10, 8); } }
Xung đo tại chân PB8:
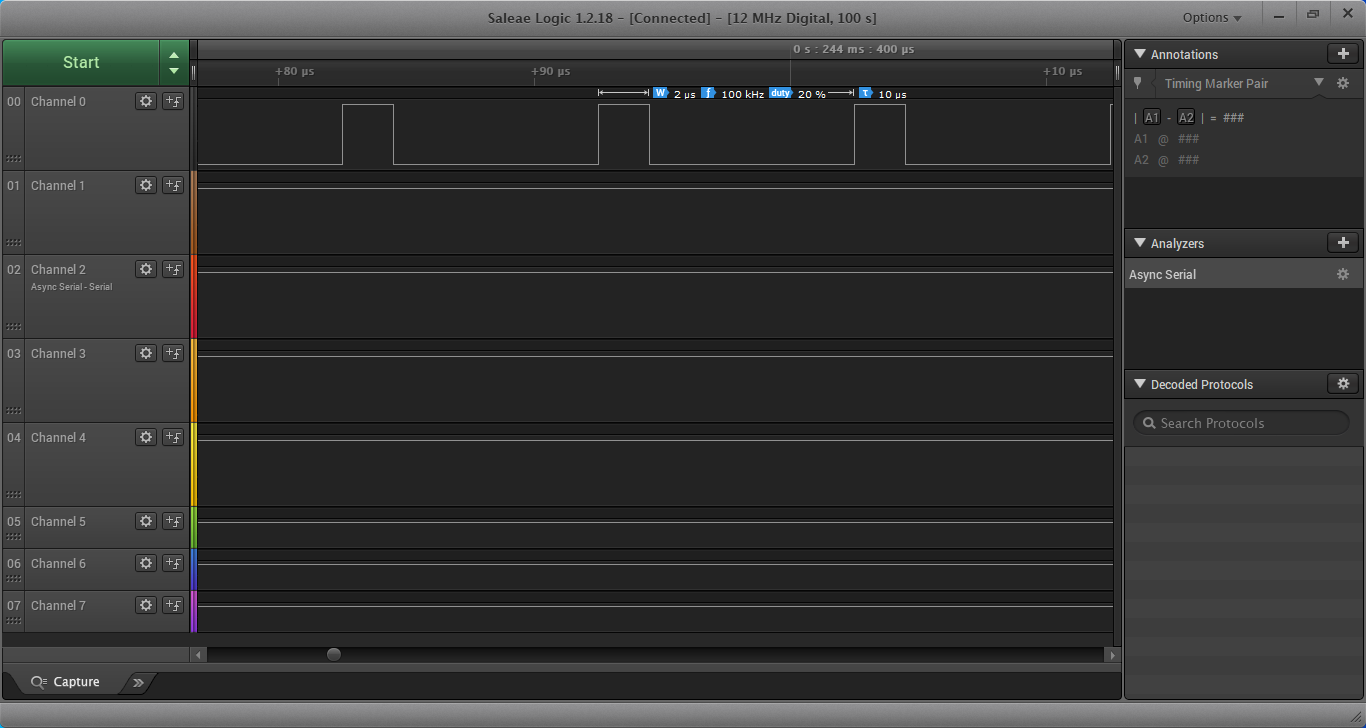
Mình thêm 1 đoạn hàm vào, hiện tại hàm này không làm gì cả, và thực hiện lời gọi tới hàm này
void test(uint8_t *pu8Data); void test(uint8_t *pu8Data) { }
Đoạn code trở thành như sau:
#include "stm32f4xx.h" // Device header void delay_ms(uint32_t u32DelayInMs); void delay_ms(uint32_t u32DelayInMs) { while (u32DelayInMs) { TIM_SetCounter(TIM6, 0); while (TIM_GetCounter(TIM6) < 1000) { } --u32DelayInMs; } } void test(uint8_t *pu8Data); void test(uint8_t *pu8Data) { } uint8_t u8Data[4]; int main(void) { GPIO_InitTypeDef gpioInit; TIM_TimeBaseInitTypeDef timInit; TIM_OCInitTypeDef pwmInit; RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOD, ENABLE); gpioInit.GPIO_Mode = GPIO_Mode_OUT; gpioInit.GPIO_OType = GPIO_OType_PP; gpioInit.GPIO_Pin = GPIO_Pin_12; gpioInit.GPIO_PuPd = GPIO_PuPd_NOPULL; gpioInit.GPIO_Speed = GPIO_High_Speed; GPIO_Init(GPIOD, &gpioInit); RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM6, ENABLE); timInit.TIM_Prescaler = 84 - 1; timInit.TIM_Period = 0xFFFF; TIM_TimeBaseInit(TIM6, &timInit); TIM_Cmd(TIM6, ENABLE); /* timer 10 */ RCC_APB2PeriphClockCmd(RCC_APB2Periph_TIM10, ENABLE); timInit.TIM_Prescaler = 168 - 1; timInit.TIM_Period = 10 - 1; TIM_TimeBaseInit(TIM10, &timInit); pwmInit.TIM_OCMode = TIM_OCMode_PWM1; pwmInit.TIM_OCPolarity = TIM_OCPolarity_High; pwmInit.TIM_Pulse = 2; pwmInit.TIM_OutputState = TIM_OutputState_Enable; TIM_OC1Init(TIM10, &pwmInit); RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOB, ENABLE); gpioInit.GPIO_Mode = GPIO_Mode_AF; gpioInit.GPIO_OType = GPIO_OType_PP; gpioInit.GPIO_Pin = GPIO_Pin_8; gpioInit.GPIO_PuPd = GPIO_PuPd_NOPULL; gpioInit.GPIO_Speed = GPIO_High_Speed; GPIO_Init(GPIOB, &gpioInit); GPIO_PinAFConfig(GPIOB, GPIO_PinSource8, GPIO_AF_TIM10); TIM_Cmd(TIM10, ENABLE); test(u8Data); while (1) { GPIO_SetBits(GPIOD, GPIO_Pin_12); delay_ms(100); //TIM_SetCompare1(TIM10, 4); GPIO_ResetBits(GPIOD, GPIO_Pin_12); delay_ms(200); //TIM_SetCompare1(TIM10, 8); } }
Và kết quả là sau khi nạp chương trình, led vẫn nháy nhưng không có xung PWM???
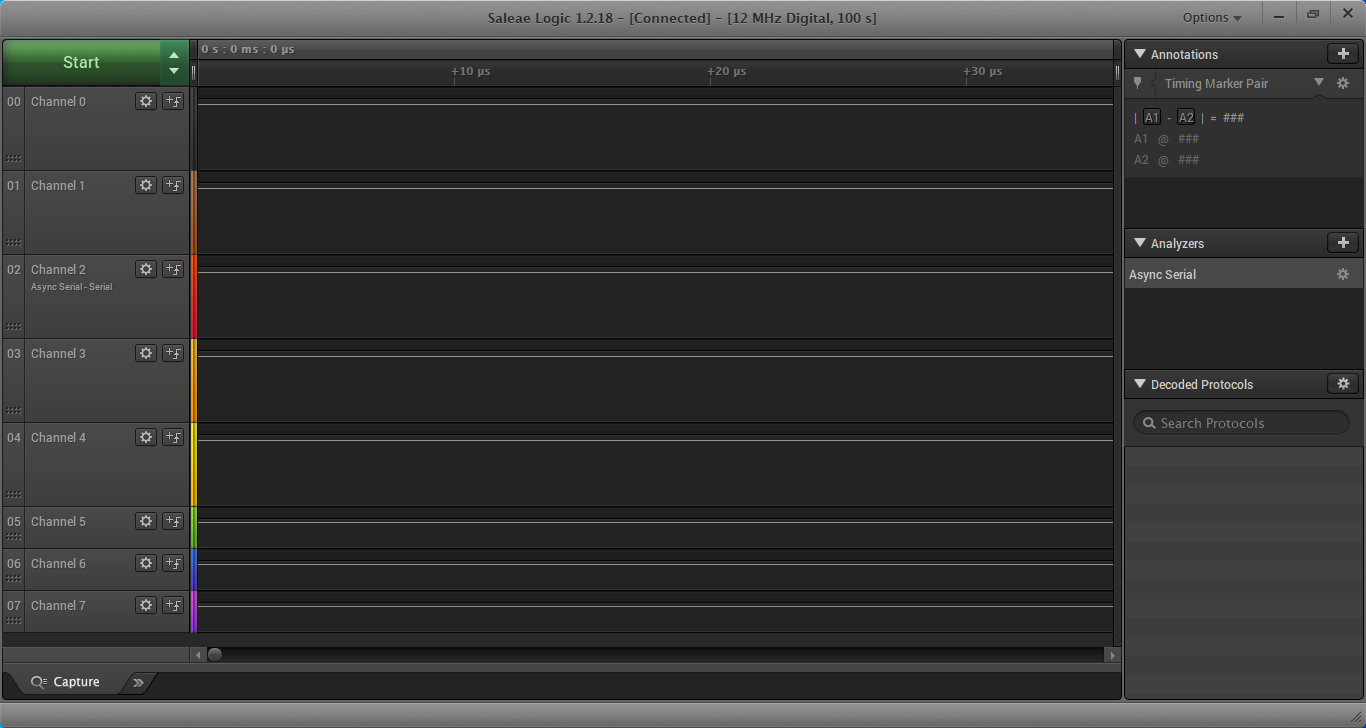
Mình gửi lên cả 2 project.
Mọi người cùng thảo luận, tìm hiểu xem lý do tại sao?
https://drive.google.com/open?id=1ZA9L4kQdYuviesuxUQ_AZqibQbNM4Era
https://drive.google.com/open?id=17X9soYrPG_6wQhrpafBY5tgfWyL5GVuD
Update:
Mình đã thử thêm với timer 11, timer 13, timer 14 kết quả đều như vậy:
Timer 11 – PWM chân PB9
https://drive.google.com/open?id=1FSgMw1LNmn930gWmYlsgb9UhBwFFBTVa
Timer 13 – PWM chân PA6
https://drive.google.com/open?id=16kiGaRibwCkFpx5Vlnmob0xlEoi_pmEm
Timer 14 – PWM chân PA7
https://drive.google.com/open?id=1DSQ7Haiu16ZvV0qyem88c9I9Na4Q4gIV
Tất cả đều hoạt động bình thường nếu chuyển dòng số 73 gọi hàm test(u8Data); thành comment.